mojo's Blog
리스트뷰와 그리드뷰 본문
리스트뷰
리스트뷰(ListView)는 데이터를 리스트 모양으로 보여주며 리스트 중 하나를 선택하는 용도로 사용한다.
activity_main.xml Code
java
닫기<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<ListView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/listView1"/>
</LinearLayout>
MainActivity.java Code
java
닫기public class MainActivity extends AppCompatActivity {
ListView list;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
setTitle("연습하기");
final String[] mid = {"고양이", "개", "라이언", "토끼", "비둘기", "구구구"};
list = (ListView)findViewById(R.id.listView1);
ArrayAdapter<String> adapter = new ArrayAdapter<String>(this,
android.R.layout.simple_list_item_multiple_choice, mid);
list.setChoiceMode(ListView.CHOICE_MODE_MULTIPLE);
list.setAdapter(adapter);
list.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> adapterView, View view, int i, long l) {
Toast.makeText(getApplicationContext(), mid[i], Toast.LENGTH_SHORT).show();
}
});
}
}
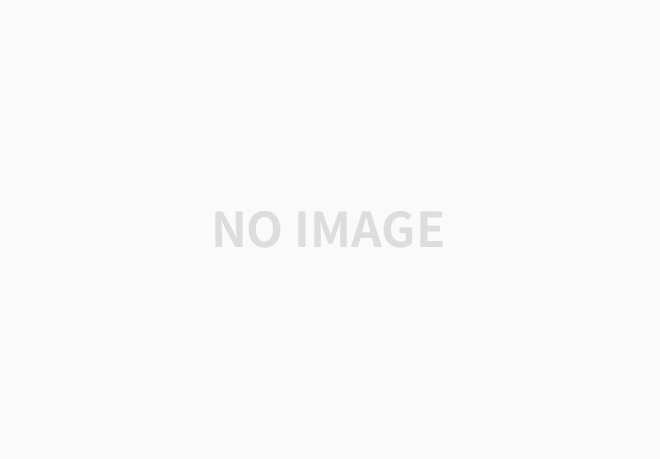
여기서 라디오 버튼으로 변경하려면 CHOICE_MODE_SINGLE 을 사용한다.
리스트뷰의 동적 추가와 삭제하는 코드
이때 추가는 항목 추가를 누르면 추가되고 삭제는 해당 목록에 있는 임의의 것을 길게 누르면 삭제된다.
activity_main.xml Code
java
닫기<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="10dp"
android:orientation="vertical">
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="입력하세요"
android:id="@+id/edit1"/>
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/btn1"
android:text="항목 추가"/>
<ListView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/listView1"/>
</LinearLayout>
MainActivity.java Code
java
닫기public class MainActivity extends AppCompatActivity {
EditText edit;
Button btn;
ListView list;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
setTitle("연습하기");
edit = (EditText)findViewById(R.id.edit1);
btn = (Button)findViewById(R.id.btn1);
list = (ListView)findViewById(R.id.listView1);
final ArrayList<String> midList = new ArrayList<String>();
final ArrayAdapter<String> adapter = new ArrayAdapter<String>(this, android.R.layout.simple_list_item_1, midList);
list.setAdapter(adapter);
btn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
String s = edit.getText().toString();
midList.add(s);
adapter.notifyDataSetChanged();
}
});
list.setOnItemLongClickListener(new AdapterView.OnItemLongClickListener() {
@Override
public boolean onItemLongClick(AdapterView<?> adapterView, View view, int i, long l) {
midList.remove(i);
adapter.notifyDataSetChanged();
return false;
}
});
}
}
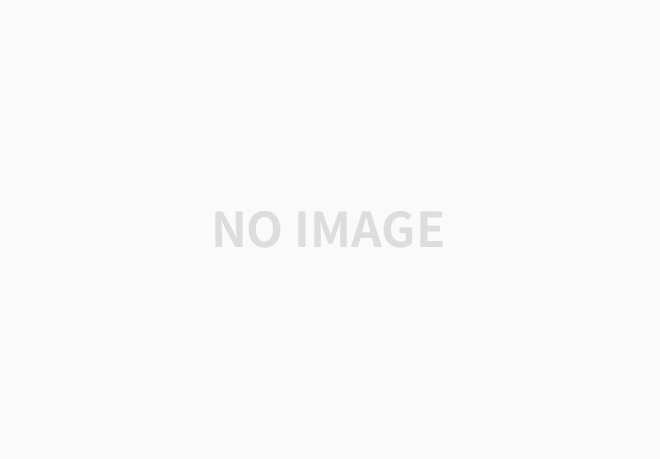
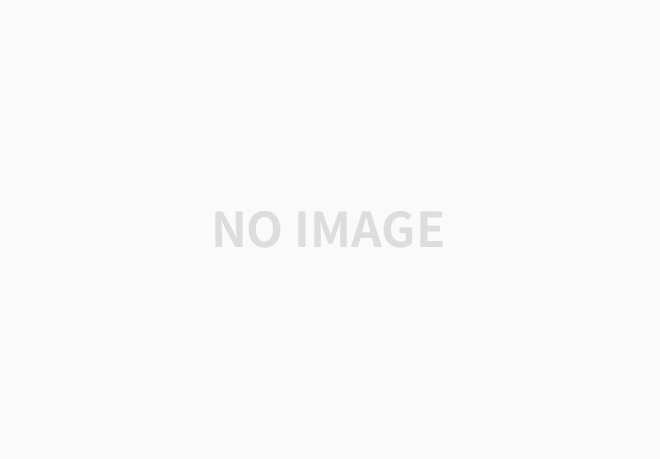
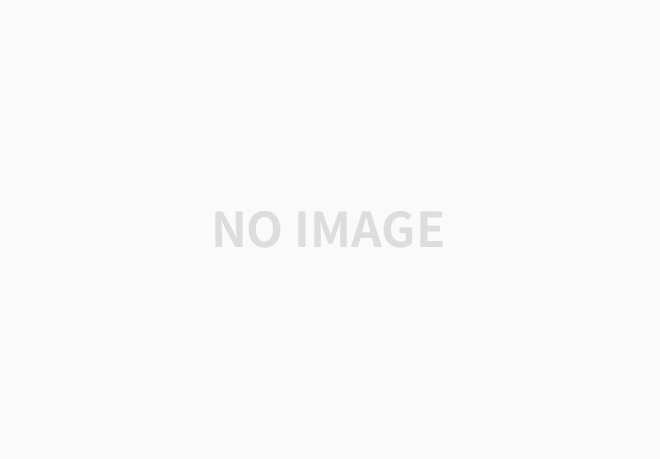
그리드뷰
그리드뷰는 사진이나 그림을 격자 모양으로 배치해준다.
버튼이나 텍스트 등을 배치할 수 있지만 주로 사진이나 그림을 배치한다.
연습하기 ) 이미지 포스터를 볼 수 있는 코드 작성하기
activity_main.xml Code
java
닫기<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="10dp"
android:orientation="vertical">
<GridView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/gridView1"
android:gravity="center"
android:numColumns="4"/>
</LinearLayout>
dialog1.xml Code
java
닫기<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical" android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center">
<ImageView
android:layout_width="150dp"
android:layout_height="150dp"
android:scaleType="fitCenter"
android:id="@+id/img1"/>
</LinearLayout>
MainActivity.java Code
java
닫기public class MainActivity extends AppCompatActivity {
Integer []moveId = {R.drawable.oreo, R.drawable.lion, R.drawable.kitty,
R.drawable.rabbit, R.drawable.cherry, R.drawable.apple,
R.drawable.dog, R.drawable.cat, R.drawable.bear,
R.drawable.oreo, R.drawable.lion, R.drawable.kitty,
R.drawable.rabbit, R.drawable.cherry, R.drawable.apple,
R.drawable.dog, R.drawable.cat, R.drawable.bear,
R.drawable.oreo, R.drawable.lion, R.drawable.kitty,
R.drawable.rabbit, R.drawable.cherry, R.drawable.apple,
R.drawable.dog, R.drawable.cat, R.drawable.bear};
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
setTitle("연습하기");
final GridView grid;
grid = (GridView)findViewById(R.id.gridView1);
MyGridAdapter gAdapter = new MyGridAdapter(this);
grid.setAdapter(gAdapter);
}
public class MyGridAdapter extends BaseAdapter {
Context context;
public MyGridAdapter(Context c){
context = c;
}
public int getCount(){
return moveId.length;
}
public Object getItem(int arg0){
return null;
}
public long getItemId(int arg0){
return 0;
}
public View getView(int position, View arg1, ViewGroup arg2){
ImageView imageview = new ImageView(context);
imageview.setLayoutParams(new GridView.LayoutParams(200, 300));
imageview.setScaleType(ImageView.ScaleType.FIT_CENTER);
imageview.setPadding(5,5,5,5);
imageview.setImageResource(moveId[position]);
final int pos = position;
imageview.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Toast.makeText(getApplicationContext(),"클릭함",Toast.LENGTH_SHORT).show();
View dialogView = (View)View.inflate(MainActivity.this, R.layout.dialog1, null);
AlertDialog.Builder dlg = new AlertDialog.Builder(MainActivity.this);
ImageView imgMove = (ImageView)dialogView.findViewById(R.id.img1);
imgMove.setImageResource(moveId[pos]);
dlg.setTitle("큰 포스터");
dlg.setIcon(R.drawable.ic_launcher_background);
dlg.setView(dialogView);
dlg.setNegativeButton("닫기",null);
dlg.show();
}
});
return imageview;
}
}
}
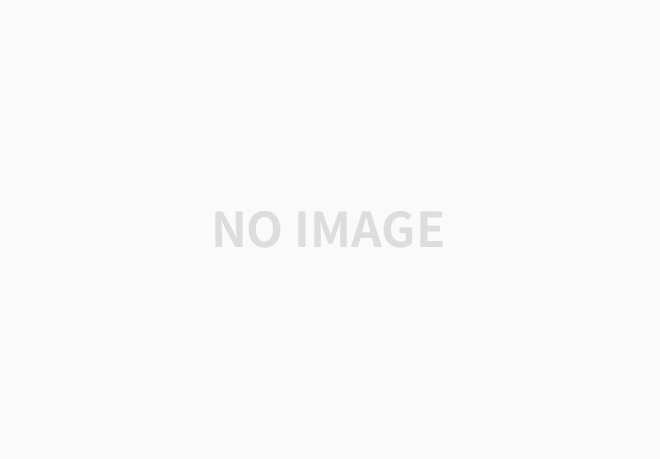
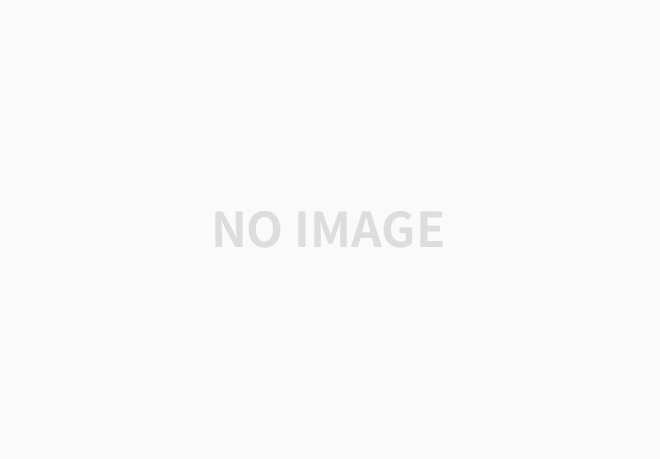
'Android' 카테고리의 다른 글
데이터베이스 기본 개념 알아가기 (0) | 2021.09.05 |
---|---|
갤러리와 스피너 (0) | 2021.09.05 |
안드로이드 프로그래밍 제 10장 연습문제 6번 (0) | 2021.09.04 |
액티비티와 인텐트 응용 (0) | 2021.09.04 |
액티비티와 인텐트의 기본 (0) | 2021.09.04 |
Comments