mojo's Blog
Graphic 본문
안드로이드는 화면에 선, 원, 사각형 드으이 도형을 그리는 방식을 제공한다.
관련 메소드에서 좌표를 직접 입력해서 그릴 수도 있고, 화면을 손가락으로 터치해서 그릴 수도 있다.
그리고 그래픽을 처리하는 방식을 잘 활용하면 그림판 같은 유용한 앱을 만들 수 있다.
그래픽 처리의 기본
그래픽을 출력할 때는 주로 View.onDraw() 메소드를 오버라이딩해서 사용한다.
그래픽 처리를 위한 기본적인 방식을 알아보도록 한다.
Java Code
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(new MyGraphicView(this));
setTitle("연습하기");
}
private static class MyGraphicView extends View{
public MyGraphicView(Context context){
super(context);
}
protected void onDraw(Canvas canvas){
super.onDraw(canvas);
Paint paint = new Paint();
paint.setAntiAlias(true);
paint.setColor(Color.GREEN);
canvas.drawLine(10,10,300,10,paint);
paint.setColor(Color.BLUE);
paint.setStrokeWidth(5);
canvas.drawLine(10,30,300,30,paint);
paint.setColor(Color.RED);
paint.setStrokeWidth(0);
paint.setStyle(Paint.Style.FILL);
Rect rect1 = new Rect(10,50,10+100,50+100);
canvas.drawRect(rect1, paint);
paint.setStyle(Paint.Style.STROKE);
Rect rect2 = new Rect(130,50,130+100,50+100);
canvas.drawRect(rect2,paint);
RectF rect3 = new RectF(250,50,250+100,50+100);
canvas.drawRoundRect(rect3,20,20,paint);
canvas.drawCircle(60,220,50,paint);
paint.setStrokeWidth(5);
Path path1 = new Path();
path1.moveTo(10,290);
path1.lineTo(10+50,290+50);
path1.lineTo(10+100,290);
path1.lineTo(10+150,290+50);
path1.lineTo(10+200,290);
canvas.drawPath(path1, paint);
paint.setStrokeWidth(0);
paint.setTextSize(30);
canvas.drawText("Android",10,390,paint);
}
}
}
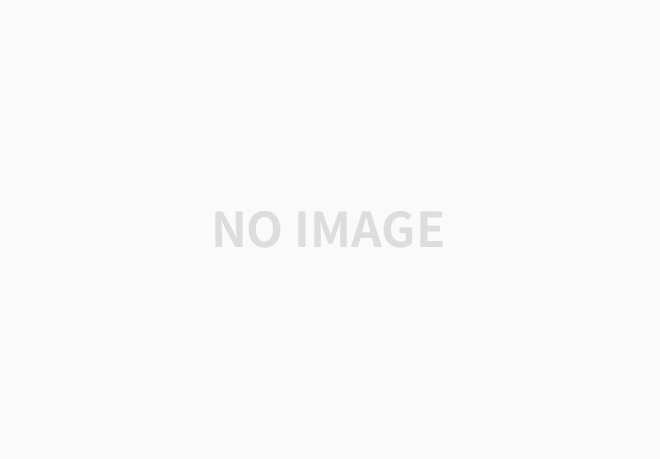
paint.setAntiAlias(true); => 도형의 끝을 부드럽게 처리해 준다.
paint.setStrokeWidth(5); => 그려질 도형 또는 글자 외곽선의 두께를 결정한다.
연습하기 ) 다음 화면이 나오도록 Java coding
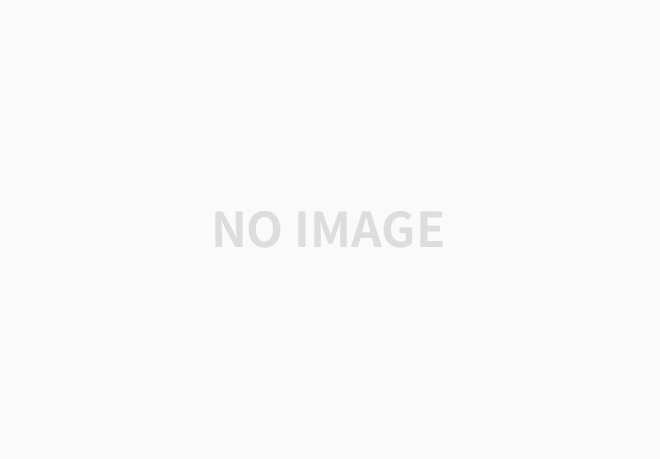
Java 코드
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(new MyGraphicView(this));
setTitle("연습하기");
}
private static class MyGraphicView extends View{
public MyGraphicView(Context context){
super(context);
}
protected void onDraw(Canvas canvas){
super.onDraw(canvas);
Paint paint = new Paint();
paint.setStrokeWidth(20);
paint.setStrokeCap(Paint.Cap.SQUARE);
paint.setColor(Color.BLACK);
canvas.drawLine(100,50,500,50,paint);
paint.setColor(Color.BLACK);
paint.setStrokeCap(Paint.Cap.ROUND);
canvas.drawLine(100,100,500,100,paint);
RectF rectF = new RectF(100,150,500,300);
canvas.drawOval(rectF,paint);
RectF rectF1 = new RectF(100,250,300,400);
canvas.drawArc(rectF1,30,120,true, paint);
paint.setColor(Color.argb(255,0,0,255));
RectF rectF2 = new RectF(100,450,300,650);
canvas.drawRect(rectF2,paint);
paint.setColor(Color.argb(100,255,0,0));
RectF rectF3 = new RectF(150,500,350,700);
canvas.drawRect(rectF3,paint);
}
}
}
Touch Event
화면에 생성한 뷰를 터치하면 Touch Event가 발생한다.
손가락으로 그림을 그리려면 터치 이벤트를 활용해야 한다.
터치를 구현하려면 View 클래스의 onTouchEvent() 메소드를 오버라이딩하여 코딩해야 한다.
연습하기 ) 간단한 그림판 앱 만들어보기
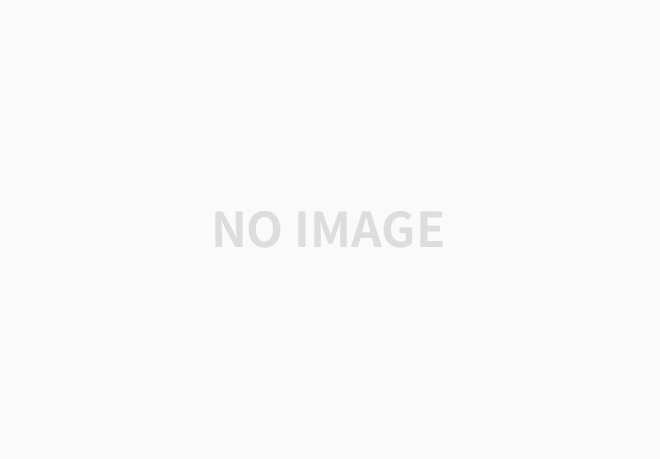
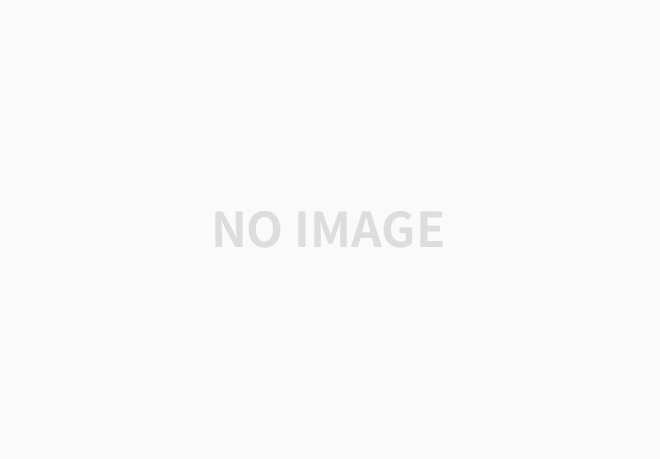
Java Code
public class MainActivity extends AppCompatActivity {
final static int LINE = 1, CIRCLE = 2;
static int curShape = LINE;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(new MyGraphicView(this));
setTitle("연습하기");
}
public boolean onCreateOptionsMenu(Menu menu){
super.onCreateOptionsMenu(menu);
menu.add(0,1,0,"선 그리기");
menu.add(0,2,0,"원 그리기");
return true;
}
public boolean onOptionsItemSelected(MenuItem item){
switch(item.getItemId()){
case 1:
curShape = LINE;
return true;
case 2:
curShape = CIRCLE;
return true;
}
return super.onOptionsItemSelected(item);
}
private static class MyGraphicView extends View{
int startX = -1, startY = -1, stopX = -1, stopY = -1;
public MyGraphicView(Context context){
super(context);
}
public boolean onTouchEvent(MotionEvent event){
switch(event.getAction()){
case MotionEvent.ACTION_DOWN:
startX = (int)event.getX();
startY = (int)event.getY();
break;
case MotionEvent.ACTION_MOVE:
case MotionEvent.ACTION_UP:
stopX = (int)event.getX();
stopY = (int)event.getY();
this.invalidate();
break;
}
return true;
}
protected void onDraw(Canvas canvas){
super.onDraw(canvas);
Paint paint = new Paint();
paint.setAntiAlias(true);
paint.setStrokeWidth(5);
paint.setStyle(Paint.Style.STROKE);
paint.setColor(Color.RED);
switch(curShape){
case LINE:
canvas.drawLine(startX, startY, stopX, stopY, paint);
break;
case CIRCLE:
int radius = (int)(Math.sqrt(Math.pow(stopX-startX, 2)+Math.pow(stopY-startY, 2)));
canvas.drawCircle(startX, startY, radius, paint);
break;
}
}
}
}
this.invalidate() 를 호출하면 화면이 무효화되고 onDraw() 메소드를 자동으로 실행하게 한다.
'Android' 카테고리의 다른 글
안드로이드 프로그래밍 제 9장 연습문제 (5, 6번) (0) | 2021.09.02 |
---|---|
Bitmap (0) | 2021.09.02 |
안드로이드 프로그래밍 제 8장 연습문제 6번 (0) | 2021.08.31 |
파일 처리 및 응용 (0) | 2021.08.31 |
안드로이드 프로그래밍 제 7장 연습문제 (4, 5, 6번) (0) | 2021.08.30 |